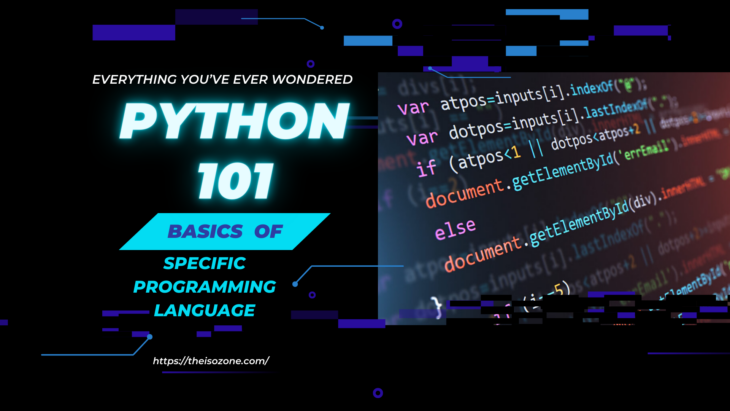
Python 101: Everything You’ve Ever Wondered, but Were Afraid to Ask
Have you ever felt like your head was spinning in circles trying to figure out all the basics of Python? Well, you’re not alone! Let’s take a deep dive into the wonderful world of Python and learn all there is to know – no questions off limits! So put on your thinking cap and let’s get started exploring all that Python has to offer.
Variables and Data Types
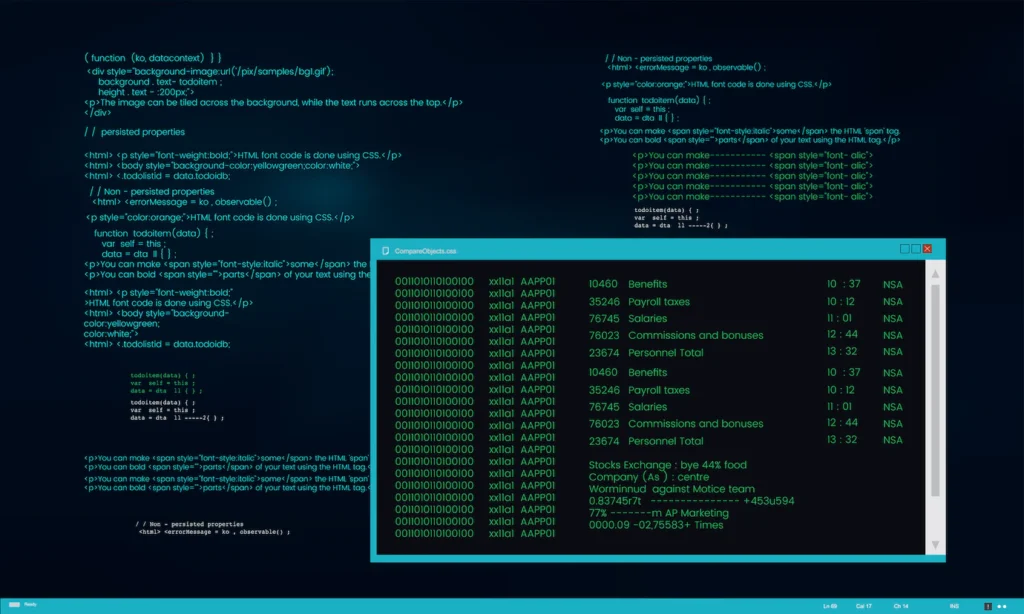
In programming, variables are used to represent pieces of data. Information that is stored in these variables can be manipulated to perform various tasks. Variables must be defined in the code before they can be used. When you define a variable, you usually assign it a value or type, known as a data type.
The types of data available vary depending on the specific programming language and there are far too many to list here, but some of the most common include Booleans, Integers, Strings and Floats. Learning variables and data types in Python 101 for beginners.
Operators and Expressions
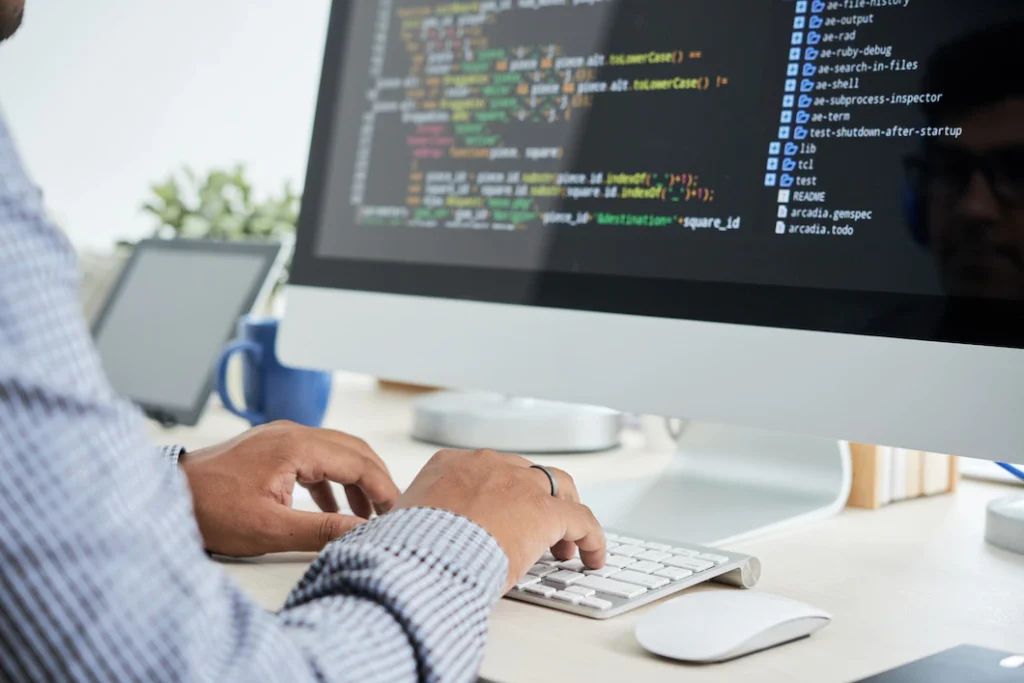
Operators are used to performing operations on variables and values. In Python, these have different levels of precedence which dictate how they are evaluated. There are seven types of operators in it: arithmetic, logical, comparison, assignment, bit-wise, membership, and identity.
• Arithmetic Operators: these are used with numeric values to perform common mathematical operations. The most commonly-used arithmetic operators in Python include add (+), subtract (-), multiply (*), divide (/), floor division (//), modulo (%) and exponent (**).
• Logical Operators: These are used to combine two or more conditions in a single expression. The three logical operators supported by Python are AND (and/&&), OR (or/||) and NOT (not/!).
• Comparison Operators: These compare two values and return either True or False based on the result of the operation. These include equality (==), inequality (!=), less than (<), greater than (>), less than or equal to (<=), and greater than or equal to (>=).
• Assignment Operators: These are used to assign a value based on a given expression. These include assignment (= ), addition assignment (+= ), subtraction assignment (-= ), multiplication assignment (*= ), and division assignment (/= ).
• Bit-wise Operators: These operate upon bits within Binary digits by performing various binary operations such as AND (&), OR (|), Exclusive OR (^ ), right shift(>>) left shift(<< ), zero-fill right shift(>>>) and unsigned right shift( >>> ).
• Membership Operators: membership operators test for membership in an object such as strings, lists, or dictionaries. The two membership Operators are in and not in.
• Identity Operators: The primary use of these is to check for the identity of two objects, not if they are equal but if they actually refer to the same object with the same memory location. The two identity operators are is & is not.
Functions
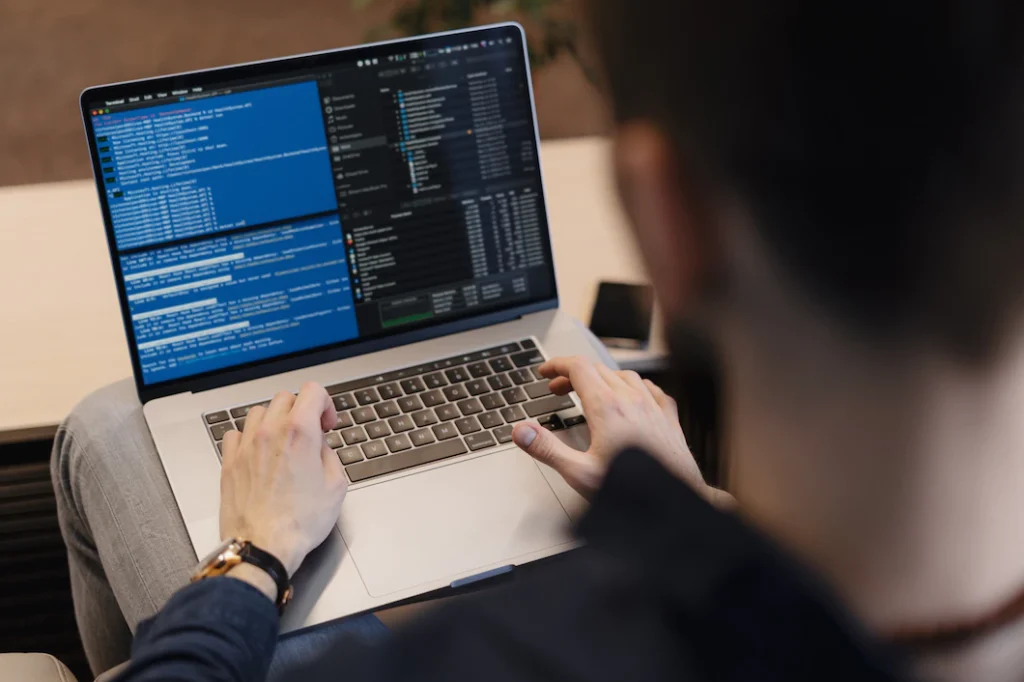
A function is a set of code that accepts input, processes it, and then provides a result or output. In Python 3, you define functions using the def keyword. After def comes the name of the function and then parentheses. Inside these parentheses maybe 0 too many parameters which are additional pieces of information provided to the function as part of its operand (data it processes).
In short, functions are extremely useful and powerful tools in Python programming because they allow us to modularize our programs into smaller, more manageable parts that can be reused again for other problems. They make debugging and updating code easier as well because all related pieces are grouped together in one place.
Working with Files
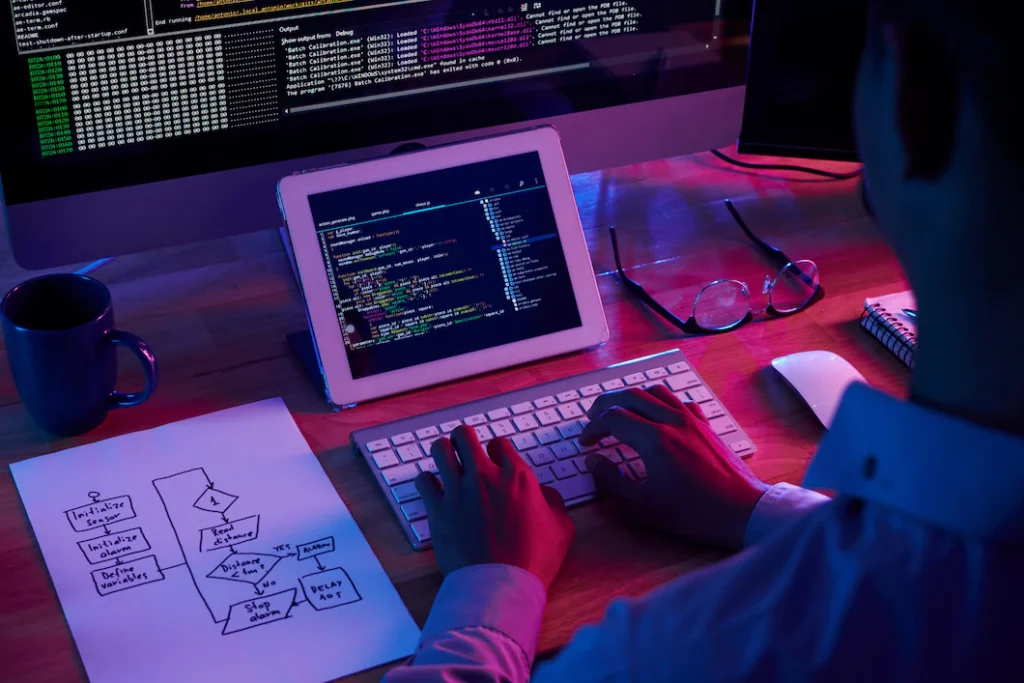
Python has several functions for interacting with the operating system and managing files. It reads and writes text files in different formats, including conventional ASCII text files and more specialized binary representations (such as pickled objects). In addition, Windows users can perform file operations from within Python using WIN32 calls.
In general then, when trying to access files from within Python many tasks will simply become a matter of appropriately manipulating an opened file object using one simple call – or multiple calls combined together over time – until you’ve accomplished your desired end result.